TSQL Latin Collation Error
Cannot resolve the collation conflict between "Latin1_General_CI_AS" and "SQL_Latin1_General_CP1_CI_AS" in the equal to operation.
I'm not sure if it's as convenient with the earlier versions but SQL Server 2008 allows you to easily change the collation of your database through the Properties window.
The first thing you would have to do is to drop all connections to the database. This can be easily done by right clicking on you database --> Tasks --> "Detach..." then tick the "Drop all connections" check box.
Then simply restore the database using your back up file (it's usually the same name as your database with a .bak file extension).
Now you're sure no one is initially connected to the database.
Right click on the database --> Properties --> Options then on the top is a drop down for the Collation options. We generally choose "SQL_Latin1_General_CP1_CI_AS".
Select XML Schema Collection type of an XML Column
I spent an afternoon looking for a way to get the XML Schema Collection type of a given column.
I wanted to copy the contents of a table (that has an XML column with a user defines Schema Collection) to another table but I get this error message: Implicit conversion between XML types constrained by different XML schema collections is not allowed. Use the CONVERT function to run this query.
I understand that I have to CONVERT it to the same XML Schema Collection but how do I do so dynamically?
I ended up resorting to MSDN's SQL forums. The next morning, Peso, an MVP, gives me the answer:
SELECT object_name(ac.object_id) AS TableName,
ac.name as ColumnName,
x.name AS CollectionName
FROM sys.all_columns AS ac
LEFT JOIN sys.xml_schema_collections AS x ON x.xml_collection_id = ac.xml_collection_id
WHERE object_name(ac.object_id) = 'MyTableNameHere'
Run SQL Script from command line
I have a 1GIG update script generated by my SQL Server Management Studio, how would you run it?
Original story: I have a perfectly running database in 2008 that has to be transferred to a 2005 server. I tried back-up and restore from 2008 to 2005 servers but that got all weird so I needed a new strategy.
Solution: Generate Script.
So I generated a script (destination: file instead of a new query window because openning a file and writing a 1Gig worth of text on it would result to a not-enough memory error). As a result, I have a 1GB update script that I can't open on the Management Studio but I have to run on 2005.
Solution: call it from the command prompt then run it.
Here's how:
1. Make sure that your script has USE Database statement at the very top. You don't have to open your file to check (actually you SHOULDN'T or your machine might hang). Just make sure you toggle the option
2. On command line type: OSQL -U [UserLogin] -i [absolutePathOfSQLscript]
For example:
OSQL -U sa -i C:\Users\Oyen\MyDocuments\1Gig_MergeScript.sql
**Note: Case matters (U != u)
You may also opt to display the script ran with the token -e which would look like: OSQL -U [UserLogin] -e -i [absolutePathOfSQLscript]
3. Password will be prompted if it is required by the login, then simply provide the password **Notice that whatever you type will not be displayed for security.
4. Press enter then wait until the script is finished running.
To note: it took me less than an hour to run a 1gigabyte update script. I hope that would help you estimate how long it will take you to run yours :)
TSQL: Unique Constraint
To add a UNIQUE constraint to a column in a table:
ALTER TABLE {TableName} ADD CONSTRAINT {ConstraintName} UNIQUE({UniqueColumnName})
Such as:
ALTER TABLE Department ADD CONSTRAINT UNIQUE_DepartmentName UNIQUE (NAME)
To add a UNIQUE constraint to two columns in a table:
ALTER TABLE {TableName} ADD CONSTRAINT {ConstraintName} UNIQUE
({UniqueColumnName1},{UniqueColumnName2})
Such as:
ALTER TABLE Department ADD CONSTRAINT UNIQUE_DepartmentNameRoom
UNIQUE(NAME,RoomNumber)
Where is aspnet_regsql.exe
But where exactly is that?Aspnet_regsql.exe file is located in the
[drive:]\%windir%\Microsoft.NET\Framework\version folder on your Web server
Here's an example of an absolute path of my aspnet_regsql.exe
C:\\Windows\Microsoft.NET\Framework\V2.0\aspnet_regsql.exe
Based on that example, it may be easier for you to determine where exactly your server's aspnet_regsql.exe file is. Be sure to take note of your server's OS (Framework vs Framework64) and its version.
TSQL Script to drop all constraints on a given table
SELECT 'ALTER TABLE ' + OBJECT_NAME(f.parent_object_id) + ' DROP CONSTRAINT ' + f.name
FROM sys.foreign_keys AS f
INNER JOIN sys.foreign_key_columns AS fc
ON f.OBJECT_ID = fc.constraint_object_id
WHERE OBJECT_NAME(f.parent_object_id) = 'MyTable'
OR OBJECT_NAME (f.referenced_object_id) = 'MyTable'
A SELECT statement that will give you the 'add constraint' queries for all constraints related to MyTable
SELECT 'ALTER TABLE ' + OBJECT_NAME(f.parent_object_id) + ' WITH CHECK ADD CONSTRAINT ' +
f.name + ' FOREIGN KEY(' + COL_NAME(fc.parent_object_id, fc.parent_column_id ) + ') REFERENCES ' + OBJECT_NAME(f.referenced_object_id) + ' (' + COL_NAME(fc.referenced_object_id,
fc.referenced_column_id) + ');'
FROM sys.foreign_keys AS f
INNER JOIN sys.foreign_key_columns AS fc
ON f.OBJECT_ID = fc.constraint_object_id
WHERE OBJECT_NAME(f.parent_object_id) = 'MyTable'
OR OBJECT_NAME (f.referenced_object_id) = 'MyTable'
TSQL: Rename Tables or Columns
EXEC sp_rename @objname='{TableName}', @newname='{NewTableName}', @objtype='TABLE'
To rename a column:
EXEC sp_rename @objname='{TableName}.{ColumnName}', @newname='{NewColumnName}', @objtype='COLUMN'
Note: you may refrain from specifying the Stored Procedure's parameters (@objname, @newname, @objtype) if you will specify the input in the proper order as specified above. Thus:
To rename a table:
EXEC sp_rename '{TableName}', '{NewTableName}', 'TABLE'
To rename a column:
EXEC sp_rename '{TableName}.{ColumnName}', '{NewColumnName}', 'COLUMN'
TSQL Error: EXECUTE permission denied on XML Column
Error we get:
System.Data.UpdateException: An error occurred while updating the entries. See the inner exception for details. ---> System.Data.SqlClient.SqlException: The EXECUTE permission was denied on the object {XMLSchema1}, database {DatabaseName}, schema 'dbo'.
Solution was simple:
GRANT EXECUTE ON XML SCHEMA COLLECTION::{XMLSchema1} TO {RestrictedLoginName}
Thanks to Bob Beauchemin.
TSQL Add/Delete User, Login, Role (Code sample)
Remember that new roles have no permissions granted on them by default. This assures that they would not be able to access certain tables, views, or stored procedures unless specifically specified.
Old, soon to be deprecated code:
DECLARE @Role varchar(20) = 'RestrictedRole'
DECLARE @DBName varchar(20) = 'DatabaseName'
DECLARE @Login varchar(120) = 'RestrictedLogin'
DECLARE @LoginPword varchar(120) = 'pazzword123'
DECLARE @counter int;
DECLARE @tablename varchar(50);
DECLARE @grantStatement nvarchar(200);
BEGIN TRY exec sp_droplogin @login; END TRY
BEGIN CATCH END CATCH
BEGIN TRY exec sp_dropuser @login; END TRY
BEGIN CATCH END CATCH
BEGIN TRY exec sp_droprole @role; END TRY
BEGIN CATCH END CATCH;
EXEC sp_addlogin @loginame = @Login
,@passwd = @LoginPword
,@defdb = @DBName;
EXEC sp_addrole @Role, 'dbo';
EXEC sp_adduser @loginame = @Login
,@grpname = @Role;
Better/more up to date code uses CREATE LOGIN instead of sys.sp_addlogin
DECLARE @Role varchar(20) = 'RestrictedRole'
DECLARE @DBName varchar(20) = 'DatabaseName'
DECLARE @Login varchar(120) = 'RestrictedLogin'
DECLARE @LoginPword varchar(120) = 'pazzword123'
DECLARE @counter int;
DECLARE @tablename varchar(50);
DECLARE @grantStatement nvarchar(200);
BEGIN TRY
SET @grantStatement = 'DROP LOGIN ' + @Login
EXEC dbo.sp_executesql @statement=@grantStatement;
END TRY
BEGIN CATCH END CATCH
BEGIN TRY
SET @grantStatement = 'DROP USER ' + @Login
EXEC dbo.sp_executesql @statement=@grantStatement;
END TRY
BEGIN CATCH END CATCH
BEGIN TRY
SET @grantStatement = 'DROP ROLE ' + @Role
EXEC dbo.sp_executesql @statement=@grantStatement;
END TRY
BEGIN CATCH END CATCH
SET @grantStatement = 'CREATE LOGIN ' + @Login + ' WITH PASSWORD = ''' + @LoginPword + ''', DEFAULT_DATABASE=' + @DBName
EXEC dbo.sp_executesql @statement=@grantStatement;
SET @grantStatement = 'CREATE ROLE ' + @Role
EXEC dbo.sp_executesql @statement=@grantStatement;
SET @grantStatement = 'CREATE USER ' + @Login + ' FOR LOGIN ' + @Login
EXEC dbo.sp_executesql @statement=@grantStatement;
Then to dynamically grant SELECT, INSERT, UPDATE, DELETE permissions on the first three tables in the current database, then append this code on the end of your choice of login/user/role creation code from above (to reuse the declared variables).
CREATE TABLE #SelectInsertUpdateTable
(
ID int IDENTITY(1,1) PRIMARY KEY
,TableName varchar(50)
)
INSERT #SelectInsertUpdateTable (TableName)
select top 3 @DBName + '.dbo.' + sys.tables.NAME from sys.tables
SET @counter = (SELECT COUNT(*) FROM #SelectInsertUpdateTable);
WHILE @counter > 0
BEGIN
SET @tablename = (SELECT TableName FROM #SelectInsertUpdateTable WHERE ID=@counter);
SET @grantStatement = 'GRANT SELECT, INSERT, UPDATE on ' + @tablename + ' to ' + @Login
EXEC dbo.sp_executesql @statement=@grantStatement;
SET @counter-=1;
END
DROP TABLE #SelectInsertUpdateTable;
TSQL Check Constraint Example
- hasSister BIT NOT NULL
- SisterName varchar(250) NULL
- SisterAge int NULL
Fill up SisterName if hasSister is true
ALTER TABLE TableName WITH CHECK ADD CONSTRAINT CK_TableName_SisterName CHECK ((hasSister=1 AND SisterName IS NOT NULL) OR (hasSister=0 AND SisterName IS NULL))Fill up SisterAge with positive int if hasSister is true
GO
ALTER TABLE TableName CHECK CONSTRAINT CK_TableName_SisterName
GO
ALTER TABLE TableName WITH CHECK ADD CONSTRAINT CK_TableName_SisterAge CHECK ((hasSister=1 AND SisterAge IS NOT NULL AND SisterAge > 0) OR (hasSister=0 AND SisterAge IS NULL))
GO
ALTER TABLE TableName CHECK CONSTRAINT CK_TableName_SisterAge
GO
simple Java switch case
I want to go through the colors in my given Color [] colors; then once it goes through all 5 colors in the array, to start all over
Best solution I can think of is using a switch case:
//define Color[] colors = {color1, color2, color3, color4, color5};
int i=0;
while(true)
{
switch (i)
{
case 1: object.setColor(color1);
break;
case 2: object.setColor(color2);
break;
case 3: object.setColor(color3);
break;
case 4: object.setColor(color4);
break;
case 5: object.setColor(color5);
break;
default: object.setColor(color1); //when all else goes wrong
break;
}
if( i == 5 )
i = 1;
else
i++;
}
where is aspnet_regsql.exe located?
It is normally located in:
C:\WINDOWS\Microsoft.NET\Framework\
Remove Identity Constraint from Column (TSQL)
In the following code, I have a PrimaryKey ID that is also set as an Identity Column. What I want to do is to remove the Identity property by creating a new column name 'tempID' then temporarily storing the ID data into it while I recreate ID column so as to remove the Identity property.
ALTER TABLE [TableName]
ADD tempID int NULL
GO
UPDATE [TableName]
SET tempID=[IdentityColumnName]
GO
-- drop all foreign key constraints related to a table
-- see TSQL Script to drop all constraints
GO
ALTER TABLE [TableName]
DROP CONSTRAINT PK_[TableName]
GO
ALTER TABLE [TableName]
DROP COLUMN [IdentityColumnName]
GO
ALTER TABLE [TableName]
ADD [IdentityColumnName] int NULL
GO
UPDATE [TableName]
SET [IdentityColumnName]=tempID
GO
ALTER TABLE [TableName]
ALTER COLUMN [IdentityColumnName] int NOT NULL
GO
ALTER TABLE [TableName]
ADD CONSTRAINT PK_[TableName] PRIMARY KEY CLUSTERED ([IdentityColumnName]) ON [PRIMARY]
--or ADD CONSTRAINT [PrimaryKeyConstraintName]
GO
-- drop all foreign key constraints related to a table
-- see TSQL Script to drop all constraints
GO
ALTER TABLE [TableName]
DROP COLUMN tempID
GO
How to drop all Database connections (TSQL)
USE master
GO
ALTER DATABASE @dbName
SET OFFLINE WITH ROLLBACK IMMEDIATE
ALTER DATABASE @dbName
SET ONLINE
OR simply
Right Click database -> Tasks -> Take database offline
How to check/count Back Links
Now let's see how to check for back links. This is useful for blogs, websites, etc.
On the Google search bar simply type
link:
For example
link:thingswelovetohate.blogspot.com
or
link:http://thingswelovetohate.blogspot.com
would result to the same number of back links.
You can determine how many back links a website has on the same window. Right above the search results, and right below the Search text box is a blue (in classic mode) highlighted line that shows how many results were found.
For example
link:www.mylot.com
You will see that it had a total of 142 back links at the time of the search.
Downgrade from SQL 2008 to SQL 2000
Detaching the database from SQL 2008 and attaching to SQL 2000 doesn't work - they tried that already. Export/Import doesn't work also - so I tried my luck on using the simplest way to do the job:
Scripting.
In SQL Server 2008 Management Studio.
Databases -> MyDatabase, right click to Tasks -> Generate Scripts.
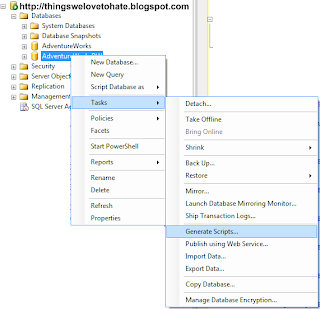
In the Script Wizard that pops up.
1. Select the database (MyDatabase/AdventureWorks)
the Check Script all objects in the selected Database.
2. Click Next.
IMPORTANT : Choose SQL 2000 in Script for Server Version
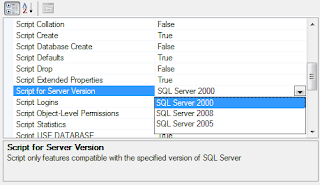
Toggle the following from False to True:
Script Database Create
Script Data
Script Indexes
Script Triggers
3. Click Next.
4. Click Finish.
Of course if you have a humongous database then the scripting the data would mean that you'd have a huge resulting .sql file. You may opt to not script the data alongside the Database itself for convenience, but if you don't have a big database then this is good enough - and pretty convenient.
How to: Inherit Database Role permissions
IF NOT EXISTS (SELECT name FROM sysusers WHERE issqlrole=1 AND name = 'RestrictedRole')
exec sp_addrole 'RestrictedRole', 'dbo';
IF NOT EXISTS (SELECT name FROM sysusers WHERE issqlrole=1 AND name = 'LessRestrictedRole')
exec sp_addrole 'LessRestrictedRole', 'dbo';
GRANT SELECT, INSERT, UPDATE on Table1 to RestrictedRole;
GRANT SELECT on Table2 to RestrictedRole;
EXEC sp_addrolemember 'RestrictedRole', 'LessRestrictedRole';
GRANT INSERT, UPDATE on Table2 to LessRestrictedRole;
The sp_addrolemember makes LessRestrictedRole a member of RestrictedRole, which means that LessRestrictedRole inherits all permissions given to RestrictedRole.
Granting more permissions to LessRestrictedRole would make those additional permissions exclusive to LessRestrictedRole - LessRestrictedRole may Insert and Update rows in Table2 while RestrictedRole may only Select rows.
By virtue of Inhertance, both LessRestrictedRole and RestrictedRole may Select, Insert and Update Table1.
CREATE new schema and ALTER table schema
To create a new Database Schema:
CREATE SCHEMA NewSchema
GO
To create a new Database Schema only if it does not exist:
IF NOT EXISTS (SELECT * FROM sys.schemas
WHERE name ='NewSchema')
EXEC dbo.sp_executesql @statement=N'
CREATE SCHEMA NewSchema';
GO
To change a table's Schema:
ALTER SCHEMA NewSchema TRANSFER OldSchema.TableName;
--default schema is dbo then:
ALTER SCHEMA NewSchema TRANSFER dbo.TableName;
Add DB Role IF NOT EXISTS
DECLARE @Role varchar(20) = 'UserRole'
IF NOT EXISTS (SELECT name FROM sysusers
WHERE issqlrole=1 AND name = @Role)
EXEC sp_addrole @Role; --EXEC sp_addrole @Role, 'dbo'
(TSQL) Escape Underscore in Like
SELECT * FROM Table WHERE Column LIKE '_a_'The above code will generate results that has any one character before and after 'a' such as:
mac
cat
sad
SELECT * FROM Table WHERE Column LIKE '%[_]a[_]%'The above code will generate results such as:
in_a_house
she_is_a_mother
make_a_living
T-SQL SELECT Random Rows in UNION
SELECT * FROM Table ORDER BY NEWID();To Select random rows from a Table UNION Table, then do:
SELECT TOP 10 PERCENT * FROM Table ORDER BY NEWID();
SELECT TOP 10 * FROM Table ORDER BY NEWID();
SELECT * FROM (SELECT TOP 10 Column1, Column2, Column 3 FROM Table1 ORDER BY NEWID()) TableA
UNION
SELECT * FROM (SELECT TOP 10 Column1, Column2, Column3 FROM Table2 ORDER BY NEWID()) TableB;
Dynamic MIME Type
But what about displaying images in Reports? We are required to provide an image type if we are to choose Database as our Image source.
This can be easily done if you an additional column of ImageName in your database alongside your Picture column. The ImageName should contain the proper extension of the image that was loaded into the database. A proper ImageName input, for example, is "megan-fox-is-a-dude.jpg".
On your Dataset query statement, include the following code:
SELECT Table.Column1, Table.Column2, Table.Column3, Table.Picture,
ISNULL(
CASE SUBSTRING(Table.ImageName,(LEN(Table.ImageName)-2),3)
WHEN 'jpg'
THEN 'image/jpeg'
ELSE 'image/' + SUBSTRING(Table.ImageName,LEN(Table.ImageName)-2,3)
END,'image/jpeg') as 'ImageExtension',
FROM Table
The CASE statement will give you the proper Image Extension based on the name of the Picture. If the Image column is NULL then it will provide a default MIMEType of 'image/jpeg' to avoid raising Errors - this is an important.
1.) Drag and drop Image to the Report Body.
2.) On the Image properties:
- set Value: =Fields!EmployeePicture.Value
- set MIMEType: =Fields!ImageExtenion.Value